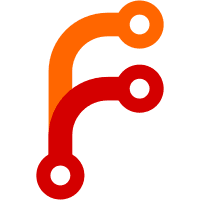
Require all implementations to provide a do_create(), a new ZephyrBinaryRunner abstract class method, and make create() itself concrete. This allows us to enforce common conventions related to individual runner capabilities as each runner provides to the core via RunnerCaps. For now, just enforce that: - common options related to capabilities are always added, so runners can't reuse them for different ends - common options provided for runners which don't support them emit sensible error messages that should be easy to diagnose and support Signed-off-by: Martí Bolívar <marti.bolivar@nordicsemi.no>
38 lines
987 B
Python
38 lines
987 B
Python
# Copyright (c) 2017 Linaro Limited.
|
|
#
|
|
# SPDX-License-Identifier: Apache-2.0
|
|
|
|
'''Runner for debugging with xt-gdb.'''
|
|
|
|
from os import path
|
|
|
|
from runners.core import ZephyrBinaryRunner, RunnerCaps
|
|
|
|
|
|
class XtensaBinaryRunner(ZephyrBinaryRunner):
|
|
'''Runner front-end for xt-gdb.'''
|
|
|
|
@classmethod
|
|
def name(cls):
|
|
return 'xtensa'
|
|
|
|
@classmethod
|
|
def capabilities(cls):
|
|
return RunnerCaps(commands={'debug'})
|
|
|
|
@classmethod
|
|
def do_add_parser(cls, parser):
|
|
parser.add_argument('--xcc-tools', required=True,
|
|
help='path to XTensa tools')
|
|
|
|
@classmethod
|
|
def do_create(cls, cfg, args):
|
|
# Override any GDB with the one provided by the XTensa tools.
|
|
cfg.gdb = path.join(args.xcc_tools, 'bin', 'xt-gdb')
|
|
return XtensaBinaryRunner(cfg)
|
|
|
|
def do_run(self, command, **kwargs):
|
|
gdb_cmd = [self.cfg.gdb, self.cfg.elf_file]
|
|
self.require(gdb_cmd[0])
|
|
self.check_call(gdb_cmd)
|